One of the challenges that often presents itself when testing modern web applications is the ubiquitous reliance on asynchronous requests embedded in the user experience. It is possible to replicate most of these actions purely using request samplers in JMeter. However, there are many cases where it is desirable to get the response for a simple task – especially where it helps us attain our main test objectives. There may also be a need to request certain out-of-context information that is vital to the test, yet not part of it. In this post, we will show you how to make dynamic requests from code using scripting elements with the JSR223 processors included with JMeter.
The JSR223 Preprocessor
JMeter includes a number of components that allow the test designer to interject Java-based code into the test plan. The JSR223 Preprocessor is one such element that can be added to run before side-by-side samplers. For example, the following preprocessor will run prior to the HTTP Request sampler that follows it:

Within the configuration panel, you can write a Groovy script to perform just about any action. Groovy is an Apache implementation of Java that is suitable for scripting tasks. Below is an example of how an independent request for a web resource from an API call could be added to a JMeter test. In the following section, we will review the code that you can customize for your particular use case.
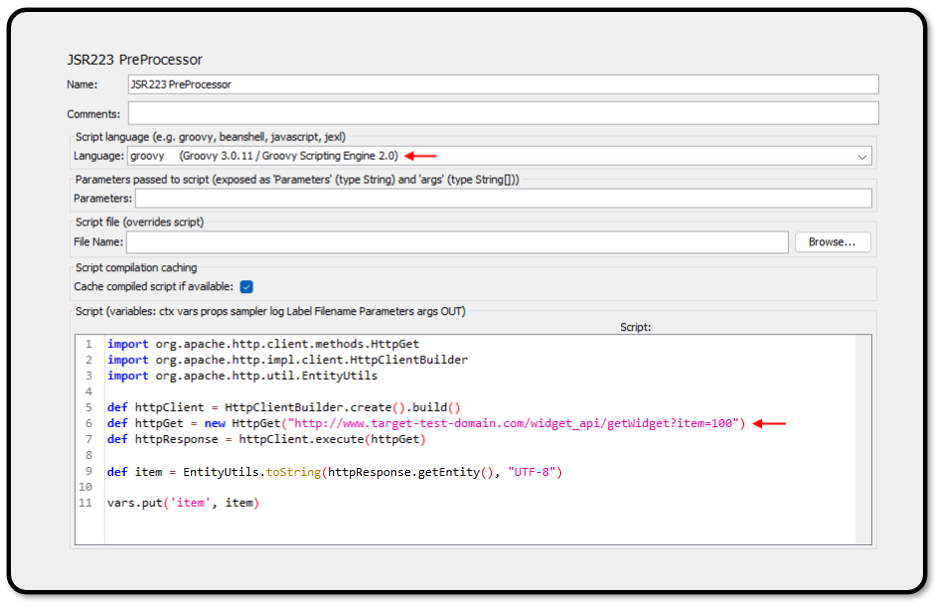
Making Direct Calls with JSR223 Components
The JSR223 Preprocessor shown above can be added anywhere in your test plan. As a preprocessor component, you will need to have an actionable element on the same level (e.g., a request sampler). Let’s use the following code example which will perform an HTTP GET request to a hypothetical API endpoint. In this case, we will make a call to a “widget API” and load the resultant JSON object into a JMeter user variable:
import org.apache.http.client.methods.HttpGet import org.apache.http.impl.client.HttpClientBuilder import org.apache.http.util.EntityUtils def httpClient = HttpClientBuilder.create().build() def httpGet = new HttpGet( "http://www.target-test-domain.com/widget_api/getWidget?item=100") def httpResponse = httpClient.execute(httpGet) def item = EntityUtils.toString(httpResponse.getEntity(), "UTF-8") vars.put('item', item)
The first few lines in the above code are import statements to load the necessary packages to make in-line web requests. You can replace the contents of HttpGet(...)
with your own web call. The last line of the script saves the expected JSON response using vars.put()
to the variable item
.
Doing Something Useful with the Result
Since the objective is to obtain out-of-context data and inject it into our test, let’s illustrate this with a basic example. In an HTTP Request that occurs after our JSR223 Preprocessor, we can use the output from our previous request to populate the POST body of another request. In the example below, we have populated another hypothetical API call to the /catalog/items
endpoint using the content from item
we previously fetched:
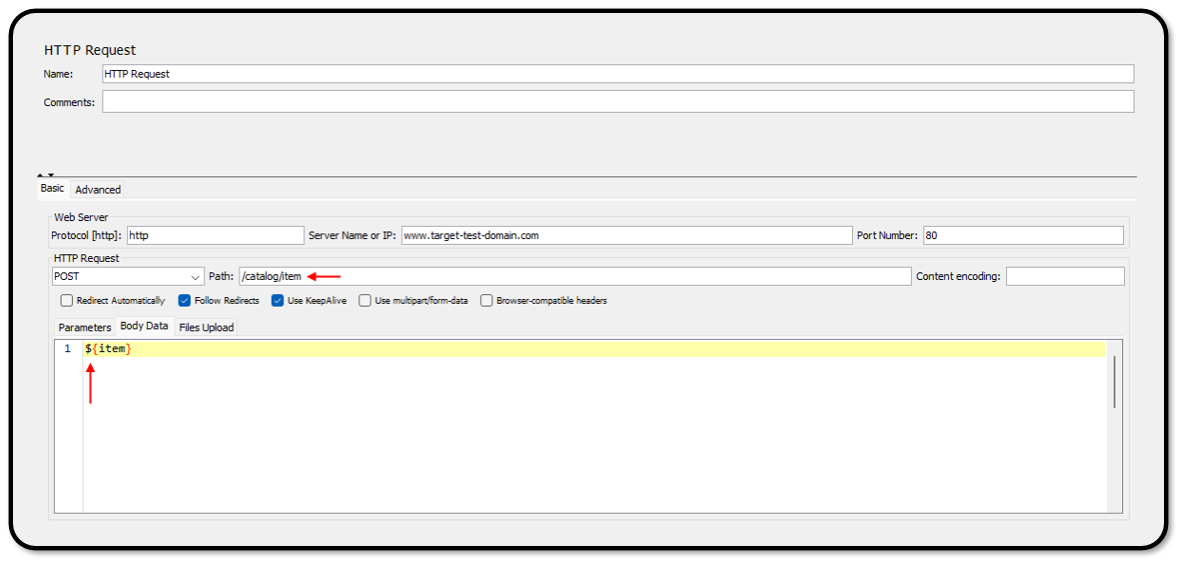
This request will execute as part of our test, and be included in the test results, unlike the request we used to populate the value for item
. Structuring your tests in this way can be useful when trying to obtain isolated results in your test output.
Other JSR223 Elements
Beyond the JSR223 Preprocessor we discussed, there are several other similar JSR223 elements that are included in JMeter. The JSR223 Postprocessor is very similar, with its key difference being scripts are executed after requests versus before them. There is even a JSR223 Sampler component which can be used in a hybrid way to the example above – and even though as a sampler the response is recorded, the scripting element provides fine control over this. You can find documentation for all of the various JSR223 elements in the official Apache JMeter component reference.
Did you know that RedLine13 offers a full-featured, time-limited free trial? Sign up now, and try the example discussed in this article for yourself today.